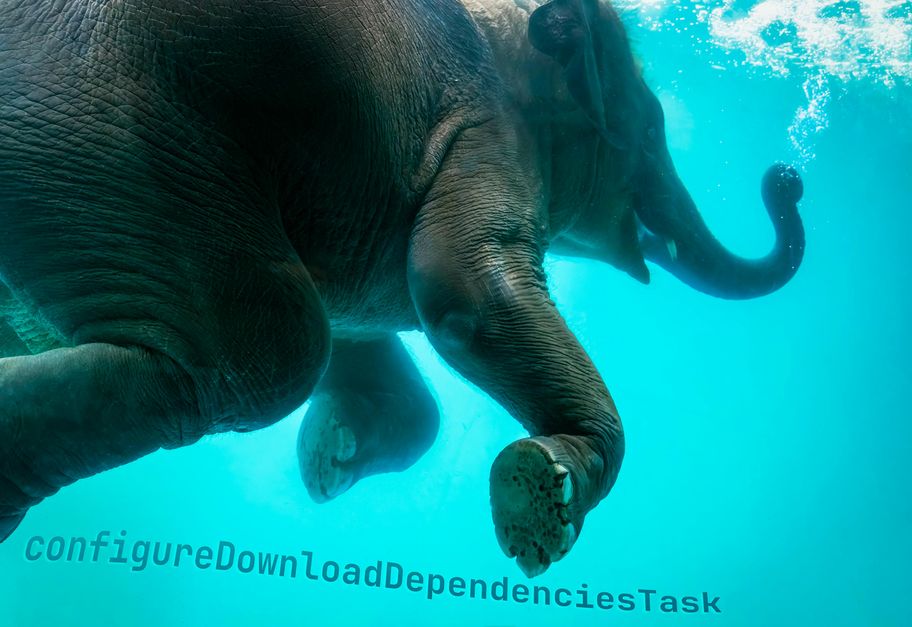
Gradle: Eagerly Get Dependencies
Table of Contents
Gradle builds need dependencies. These are libraries; the code others wrote. Your project uses them, so Gradle downloads them when needed. This can be quite time-consuming.
This script is simple. It gets all dependencies eagerly upon execution.
The Wait
You hit build. Gradle starts. It looks for dependencies. If they are not on your machine, Gradle downloads them.
Slow internet makes the wait long. Potentially, very long. This Gradle task fixes that. You run it once and all dependencies get resolved. After that, the builds won’t require additional downloads, which also allows you to work offline.
The Solution
Here is the code to put in your build.gradle.kts
or any other place you keep your build logic.
// Place this in a .kt/.kts file, for example:
// buildSrc/src/main/kotlin/DownloadDependenciesTask.kt
import org.gradle.api.Project
internal fun Project.configureDownloadDependenciesTask() =
tasks.register("downloadDependencies") {
group = "build setup"
description = "Download and cache all dependencies"
doLast {
for (config in configurations) {
if (config.isCanBeResolved) {
config.files
}
}
}
}
This tiny piece of code:
- Makes a new Gradle task:
downloadDependencies
- Resolves all configurations in the project it’s applied to. Configurations are groups of dependencies;
implementation
,testImplementation
, and others. It tries to resolve the files for each configuration. If they are not available locally, they’ll be downloaded.
Using The Script
To use this script:
Create a
kt
/kts
file.Add the code.
Apply the task in your
build.gradle.kts
file. This tells Gradle to create thedownloadDependencies
task for this project. If you have a multi-project build, you can apply it in subprojects’build.gradle.kts
files too, or in anallprojects
orsubprojects
block in the root script.Run the task: Open your terminal. Go to your project directory. Run:
./gradlew downloadDependencies
Or, on Windows:gradlew.bat downloadDependencies
Gradle will run the task. It will find all dependencies, and it will download them.
Why Bother?
This task seems small. It is, but it helps.
- Faster Builds: Once the dependencies are downloaded, they are cached. Later builds are faster. They skip the download step if the versions are the same.
- Offline Work: Run
downloadDependencies
when you have internet. Then work from anywhere. A train. A plane. A cabin in the woods. Your builds will use the cached dependencies. - CI Caching: Continuous Integration (CI) servers build your code often. Run this task early in your CI pipeline. The dependencies get cached by the CI system. Subsequent pipeline runs can be much faster.
Conclusion
The function is simple, and it saves time. It’s a simple step for a slightly better experience. Less waiting, more doing.